What are RESTful Web Services?
REST (Representational State Transfer) is a stateless client-server architecture style used for developing applications that are accessible over the web. It is a type of web service whose main goal is to make web services more effective. It can be defined as the web service that uses HTTP methods for implementing the REST architecture. Unlike SOAP which is protocol-based, Restful services are architecturally based. It does not contain any contract or WSDL file.
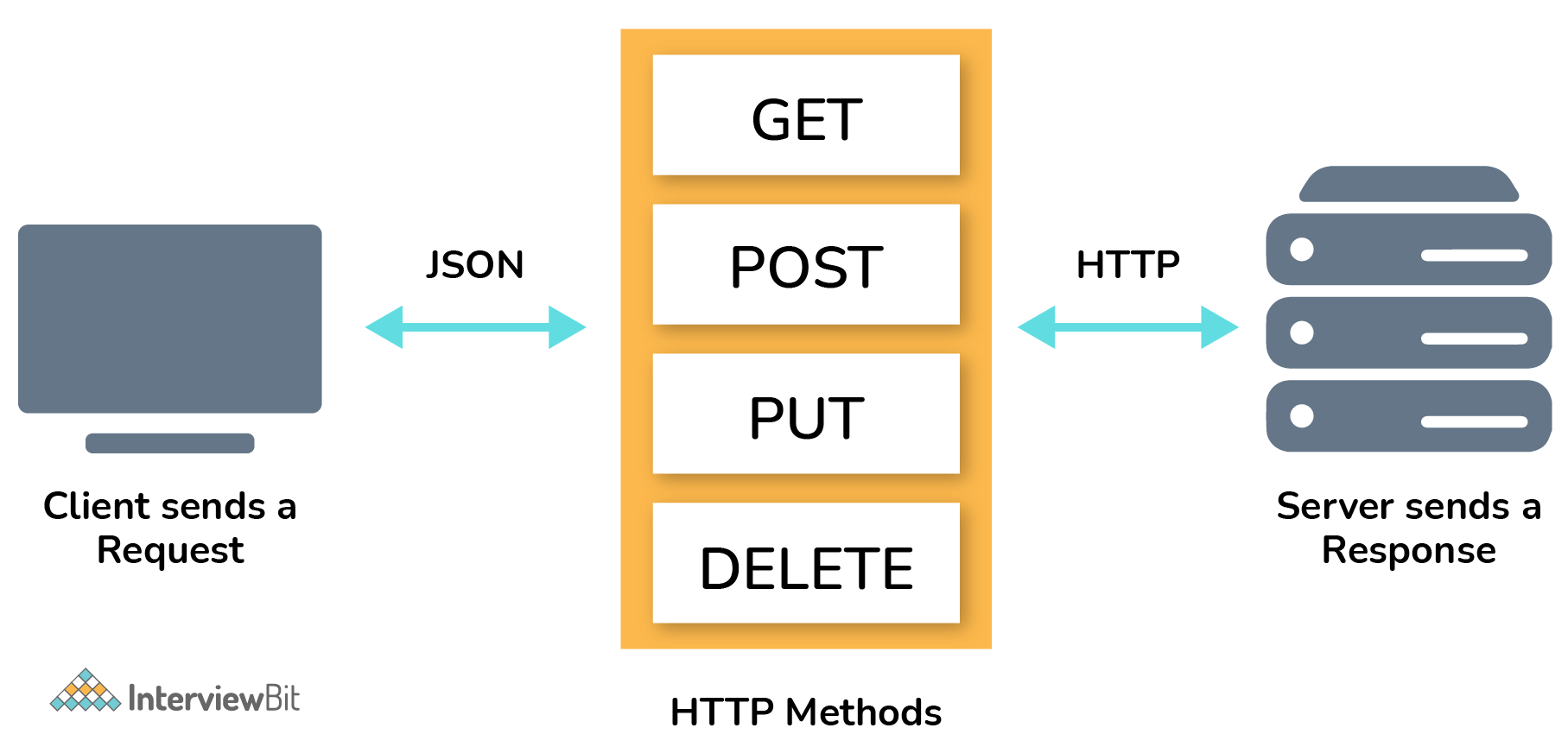
19. What are the advantages of RESTful web service?
There are several advantages of RESTful web services as given below:
- Platform independent.
- Simple and easy to implement and test.
- Support different formats such as JSON, XML, HTML, etc.
- Can be written in different programming languages and executed on any platform.
- Lightweight, manageable, scalable, and reusable.
- Faster and provide better performance.
- Consume less bandwidth and resources.
- A lot of automation framework is available
20. Which protocol is used by RESTful web services?
The protocol used by RESTful web services is HTTP.
21. Explain the term statelessness with respect to RESTful web Services. Write its advantages.
Statelessness is basically a condition or restriction where RESTful web services are not allowed to keep a client state on the server as per the REST architecture. Clients are responsible to pass their context to the server. To process the client’s request, the server then further stores this context.
Advantages:
- No need to maintain previous interactions with clients.
- Independent treatment of each method request.
- Less complexity and simplified application design.
Example: Simple GET Request using NodeJS
We have the following sample data in users.json file.
Filename: users.json
{
"user1" : {
"name" : "gourav",
"password" : "password1",
"profession" : "officer",
"id": 1
},
"user2" : {
"name" : "nikhil",
"password" : "password2",
"profession" : "teacher",
"id": 2
}
}
Implement our RESTful API listUsers using the following code in a server.js file.
Filename: server.js
// Requiring module
var express = require('express');
var app = express();
var fs = require("fs");
// Sample GET API
app.get('/listUsers', function (req, res) {
fs.readFile( __dirname + "/" + "users.json", 'utf8', function (err, data) {
console.log( data );
res.end( data );
});
})
// Server setup
var server = app.listen(8081, function () {
var host = server.address().address
var port = server.address().port
console.log("Example app listening at http://%s:%s", host, port)
})
Now open a browser and go to http://127.0.0.1:8081/listUsers, we will get the following response:
{
"user1" : {
"name" : "gourav",
"password" : "password1",
"profession" : "officer",
"id": 1
},
"user2" : {
"name" : "nikhil",
"password" : "password2",
"profession" : "teacher",
"id": 2
}
}
22. Name HTTP methods that can be used with RESTful web services.
Some of the HTTP methods that can be used with RESTful web services include:
- GET: Used to get and read a resource.
- POST: Used to create a new resource.
- PUT: Used to update existing resources.
- DELETE: Used to delete the resource.
- PATCH: Used to apply partial modifications to a resource.
23. What are the written status codes for REST API?
REST API generally returns the following status codes in HTTP response:
- 200 OK
- 201 Created
- 202 Accepted
- 302 Found
- 400 Bad Request
- 401 Unauthorized
- 404 Not Found
- 405 Method Not Allowed
- 409 Conflict
- 500 Internal Server Error
No comments:
Post a Comment