Inheritance
When one object acquires all the properties and behaviors of a parent object, it is known as inheritance. It provides code reusability. It is used to achieve runtime polymorphism.
Polymorphism
If one task is performed in different ways, it is known as polymorphism. For example: to convince the customer differently, to draw something, for example, shape, triangle, rectangle, etc.
In Java, we use method overloading and method overriding to achieve polymorphism.
Abstraction
Hiding internal details and showing functionality is known as abstraction. For example phone call, we don't know the internal processing.
In Java, we use abstract class and interface to achieve abstraction.
Encapsulation
Binding (or wrapping) code and data together into a single unit are known as encapsulation. For example, a capsule, it is wrapped with different medicines.
A java class is the example of encapsulation. Java bean is the fully encapsulated class because all the data members are private here.
======================================================
Java HashMap
Java HashMap class implements the Map interface which allows us to store key and value pair, where keys should be unique. If you try to insert the duplicate key, it will replace the element of the corresponding key. It is easy to perform operations using the key index like updation, deletion, etc. HashMap class is found in the java.util package.
Points to remember
- Java HashMap contains values based on the key.
- Java HashMap contains only unique keys.
- Java HashMap may have one null key and multiple null values.
- Java HashMap is non synchronized.
- Java HashMap maintains no order.
- The initial default capacity of Java HashMap class is 16 with a load factor of 0.75
Internal Working of HashMap
Before understanding the internal working of HashMap, you must be aware of hashCode() and equals() method.
- equals(): It checks the equality of two objects. It compares the Key, whether they are equal or not. It is a method of the Object class. It can be overridden. If you override the equals() method, then it is mandatory to override the hashCode() method.
- hashCode(): This is the method of the object class. It returns the memory reference of the object in integer form. The value received from the method is used as the bucket number. The bucket number is the address of the element inside the map. Hash code of null Key is 0.
- Buckets: Array of the node is called buckets. Each node has a data structure like a LinkedList. More than one node can share the same bucket. It may be different in capacity.
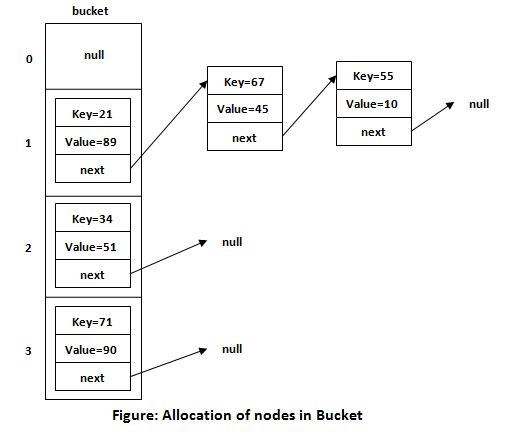
- 1. Initial Capacity – It
is the capacity of HashMap at the time of its creation (It is the number
of buckets a HashMap can hold when the HashMap is instantiated). In java,
it is 2^4=16 initially, meaning it can hold 16 key-value pairs.
- 2. Load Factor – It
is the percent value of the capacity after which the capacity of Hashmap
is to be increased (It is the percentage fill of buckets after which
Rehashing takes place). In java, it is 0.75f by default, meaning the
rehashing takes place after filling 75% of the capacity.
- 3. Threshold – It
is the product of Load Factor and Initial Capacity. In java, by default,
it is (16 * 0.75 = 12). That is, Rehashing takes place after inserting 12
key-value pairs into the HashMap.
- 4. Rehashing – It
is the process of doubling the capacity of the HashMap after it reaches
its Threshold. In java, HashMap continues to rehash(by default) in the
following sequence – 2^4, 2^5, 2^6, 2^7, …. so on.
Example
In the following example, we want to insert three (Key, Value) pair in the HashMap.
Let's see at which index the Key, value pair will be saved into HashMap. When we call the put() method, then it calculates the hash code of the Key "Aman." Suppose the hash code of "Aman" is 2657860. To store the Key in memory, we have to calculate the index.
Calculating Index
Index minimizes the size of the array. The Formula for calculating the index is:
Where n is the size of the array. Hence the index value for "Aman" is:
The value 4 is the computed index value where the Key and value will store in HashMap.
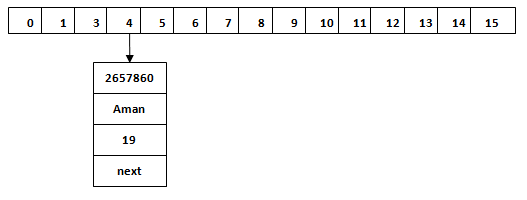
Hash Collision
This is the case when the calculated index value is the same for two or more Keys. Let's calculate the hash code for another Key "Sunny." Suppose the hash code for "Sunny" is 63281940. To store the Key in the memory, we have to calculate index by using the index formula.
The value 4 is the computed index value where the Key will be stored in HashMap. In this case, equals() method check that both Keys are equal or not. If Keys are same, replace the value with the current value. Otherwise, connect this node object to the existing node object through the LinkedList. Hence both Keys will be stored at index 4.
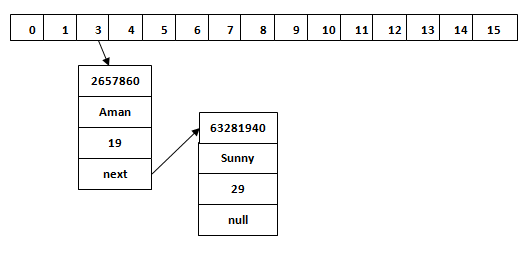
Similarly, we will store the Key "Ritesh." Suppose hash code for the Key is 2349873. The index value will be 1. Hence this Key will be stored at index 1.
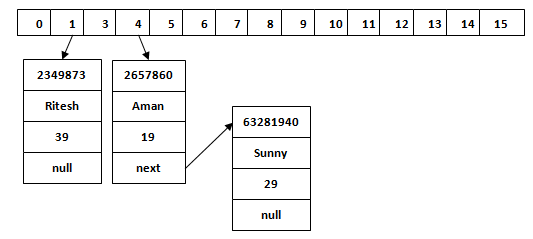
Java ConcurrentHashMap class
A hash table supporting full concurrency of retrievals and high expected concurrency for updates. This class obeys the same functional specification as Hashtable and includes versions of methods corresponding to each method of Hashtable. However, even though all operations are thread-safe, retrieval operations do not entail locking, and there is not any support for locking the entire table in a way that prevents all access. This class is fully interoperable with Hashtable in programs that rely on its thread safety but not on its synchronization details..
========================================================
Difference between Comparable and Comparator
Comparable and Comparator both are interfaces and can be used to sort collection elements.
However, there are many differences between Comparable and Comparator interfaces that are given below.
Comparable | Comparator |
---|---|
1) Comparable provides a single sorting sequence. In other words, we can sort the collection on the basis of a single element such as id, name, and price. | The Comparator provides multiple sorting sequences. In other words, we can sort the collection on the basis of multiple elements such as id, name, and price etc. |
2) Comparable affects the original class, i.e., the actual class is modified. | Comparator doesn't affect the original class, i.e., the actual class is not modified. |
3) Comparable provides compareTo() method to sort elements. | Comparator provides compare() method to sort elements. |
4) Comparable is present in java.lang package. | A Comparator is present in the java.util package. |
5) We can sort the list elements of Comparable type by Collections.sort(List) method. | We can sort the list elements of Comparator type by Collections.sort(List, Comparator) method. |
Java Comparable Example
Let's see the example of a Comparable interface that sorts the list elements on the basis of age.
Java Comparator Example
Let's see an example of the Java Comparator interface where we are sorting the elements of a list using different comparators.
Student.java
AgeComparator.java
NameComparator.java
This class provides comparison logic based on the name. In such case, we are using the compareTo() method of String class, which internally provides the comparison logic.
TestComparator.java
In this class, we are printing the values of the object by sorting on the basis of name and age.
Object class in Java
The Object class is the parent class of all the classes in java by default. In other words, it is the topmost class of java.
The Object class is beneficial if you want to refer any object whose type you don't know. Notice that parent class reference variable can refer the child class object, know as upcasting.
Let's take an example, there is getObject() method that returns an object but it can be of any type like Employee,Student etc, we can use Object class reference to refer that object. For example:
The Object class provides some common behaviors to all the objects such as object can be compared, object can be cloned, object can be notified etc.
Methods of Object class
The Object class provides many methods. They are as follows: |
Method | Description |
---|---|
public final Class getClass() | returns the Class class object of this object. The Class class can further be used to get the metadata of this class. |
public int hashCode() | returns the hashcode number for this object. |
public boolean equals(Object obj) | compares the given object to this object. |
protected Object clone() throws CloneNotSupportedException | creates and returns the exact copy (clone) of this object. |
public String toString() | returns the string representation of this object. |
public final void notify() | wakes up single thread, waiting on this object's monitor. |
public final void notifyAll() | wakes up all the threads, waiting on this object's monitor. |
public final void wait(long timeout)throws InterruptedException | causes the current thread to wait for the specified milliseconds, until another thread notifies (invokes notify() or notifyAll() method). |
public final void wait(long timeout,int nanos)throws InterruptedException | causes the current thread to wait for the specified milliseconds and nanoseconds, until another thread notifies (invokes notify() or notifyAll() method). |
public final void wait()throws InterruptedException | causes the current thread to wait, until another thread notifies (invokes notify() or notifyAll() method). |
protected void finalize()throws Throwable | is invoked by the garbage collector before object is being garbage collected. |
=========================================================
Advantage & Disadvantage of Singleton Pattern
Advantages of a Singleton pattern:
- Singleton pattern can be implemented interfaces.
- It can be also inherit from other classes.
- It can be lazy loaded.
- It has Static Initialization.
- It can be extended into a factory pattern.
- It help to It hide dependencies.
- It provides a single point if access to a particular instance, so it is easy to maintain.
Disadvantages of a Singleton Pattern
- Unit testing is more difficult (because it introduces a global state into an application).
- This pattern reduces the potential for parallelism within a program, because to access the singleton in a multi-threaded system, an object must be serialized (by locking).
Singleton class vs. Static methods
- A Static Class cannot be extended whereas a singleton class can be extended.
- A Static Class can still have instances (unwanted instances) whereas a singleton class prevents it.
- A Static Class cannot be initialized with a STATE (parameter), whereas a singleton class can be.
- A Static class is loaded automatically by the CLR when the program or namespace containing the class is loaded.
No comments:
Post a Comment